
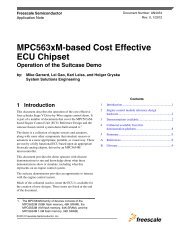
U-boot 2009.01 Driver Win 7
Im tryin to write a SPI Driver in u-boot-1.3.4 with SPI BUS 1 support for my processor AT91SAM9261 . I have defined the base macro for SPI bus 1 AT91SAM9261_BASE_SPI1 in /asm/arch/hardware.h and i av passed the bus id and chip select id . but im not getting any data or clock value on the pins . can any body let me what stupid mistake im committing? below is my source
#include <common.h>
#include <spi.h>
#include <malloc.h>
#include <asm/io.h>
#include <asm/arch/hardware.h
#include <asm/arch/at91_spi.h>
#include 'atmel_spi.h'
void spi_init()
{
/* do some initialization of SPI bus pins*/
at91_set_A_periph(AT91_PIN_PA25, 0); /* SPI0_NPCS01 */
at91_set_A_periph(AT91_PIN_PB30, 0); /* SPI0_MISO */
at91_set_A_periph(AT91_PIN_PB31, 0); /* SPI0_MOSI */
at91_set_A_periph(AT91_PIN_PB29, 0); /* SPI0_SPCK */
/* Enable clock */
at91_sys_write(AT91_PMC_PCER, 1 << AT91SAM9261_ID_SPI1);
/* Reset the SPI */
//spi_writel(ATMEL_SPI_SWRST, ATMEL_SPI_CR,1);
}
struct spi_slave *spi_setup_slave(unsigned int bus, unsigned int cs,
unsigned int max_hz, unsigned int mode)
{
struct atmel_spi_slave *as;
unsigned int scbr;
u32 csrx;
void *regs;
max_hz=15000000;/* modified for AMOLED*/
mode=ATMEL_SPI_MR_MSTR;
mode =0;/* falling edge of clock spi XFER*/
bus=AT91SAM9261_ID_SPI1;
cs=AT91_PIN_PA25;/* chip select of the slave connected to . */
if (cs > 3 || !spi_cs_is_valid(bus, cs))
return NULL;
regs = (void *)AT91SAM9261_BASE_SPI1; /* spi bus 1 ID of the */
/* Reset the SPI */
spi_writel(as,ATMEL_SPI_CR,ATMEL_SPI_SWRST);
scbr = (AT91_MASTER_CLOCK/max_hz)<<8; /* configure serial clock baud rate*/
if (scbr > ATMEL_SPI_CSRx_SCBR_MAX)
/* Too low max SCK rate */
return NULL;
if (scbr < 1)
scbr = 1;
csrx = ATMEL_SPI_CSRx_SCBR(scbr);
csrx |= ATMEL_SPI_CSRx_BITS(ATMEL_SPI_BITS_8);
if (!(mode & SPI_CPHA))
csrx |= ATMEL_SPI_CSRx_NCPHA;
if (mode & SPI_CPOL)
csrx |= ATMEL_SPI_CSRx_CPOL;
as = malloc(sizeof(struct atmel_spi_slave));
if (!as)
return NULL;
as->slave.bus = bus;
as->slave.cs = cs;
as->regs = regs;
as->mr = ATMEL_SPI_MR_MSTR | ATMEL_SPI_MR_MODFDIS
| ATMEL_SPI_MR_PCS(~(1 << cs) & 0xf);
as->mr = ATMEL_SPI_CSRx_NCPHA | ATMEL_SPI_CSR(2) | ATMEL_SPI_MR_MSTR ;/* configure chipselect*/
spi_writel(as, CSR(cs), csrx);
return &as->slave;
}
void spi_free_slave(struct spi_slave *slave)
{
struct atmel_spi_slave *as = to_atmel_spi(slave);
free(as);
}
int spi_claim_bus(struct spi_slave *slave)
{
struct atmel_spi_slave *as = to_atmel_spi(slave);
/* Enable the SPI hardware */
spi_writel(as, CR, ATMEL_SPI_CR_SPIEN);
/*
* Select the slave. This should set SCK to the correct
* initial state, etc.
*/
spi_writel(as, MR, as->mr);
return 0;
}
void spi_release_bus(struct spi_slave *slave)
{
struct atmel_spi_slave *as = to_atmel_spi(slave);
/* Disable the SPI hardware */
spi_writel(as, CR, ATMEL_SPI_CR_SPIDIS);
}
int spi_xfer(struct spi_slave *slave, unsigned int bitlen,
const void *dout, void *din, unsigned long flags)
{
struct atmel_spi_slave *as = to_atmel_spi(slave);
unsigned int len_tx;
unsigned int len_rx;
unsigned int len;
int ret;
u32 status;
unsigned short mask ;
const u8 *txp = dout;
u8 *rxp = din;
u8 value;
unsigned int pol;
bitlen=8;
ret = 0;
if (bitlen 0)
/* Finish any previously submitted transfers */
goto out;
/*
* TODO: The controller can do non-multiple-of-8 bit
* transfers, but this driver currently doesn't support it.
*
* It's also not clear how such transfers are supposed to be
* represented as a stream of bytes..this is a limitation of
* the current SPI interface.
*/
if (bitlen % 8) {
/* Errors always terminate an ongoing transfer */
flags |= SPI_XFER_END;
goto out;
}
len = bitlen / 8;
/*
* The controller can do automatic CS control, but it is
* somewhat quirky, and it doesn't really buy us much anyway
* in the context of U-Boot.
*/
if (flags & SPI_XFER_BEGIN)
spi_cs_activate(slave);
for (len_tx = 0, len_rx = 0; len_rx < len; ) {
status = spi_readl(as, SR);
if (status & ATMEL_SPI_SR_OVRES)
return -1;
if (len_tx < len && (status & ATMEL_SPI_SR_TDRE)) {
if (txp)
value = *txp++;
else
value = 0;
udelay(500000);
pol=spi_claim_bus(slave);
spi_writel(as, TDR, value); /*write data into device */
spi_release_bus(slave)
len_tx++;
if (status & ATMEL_SPI_SR_RDRF) {
value = spi_readl(as, RDR);
if (rxp)
*rxp++ = value;
len_rx++;
}
}
out:
if (flags & SPI_XFER_END) {
/*
* Wait until the transfer is completely done before
* we deactivate CS.
*/
do {
status = spi_readl(as, SR);
} while (!(status & ATMEL_SPI_SR_TXEMPTY));
//spi_writel(as,ATMEL_SPI_CSRx_CSAAT, value);
spi_cs_deactivate(slave);
}
return 0;
}

U-boot 2009.01 Driver Ed
The Das Universal Bootloader (U-Boot) is a firmware/bootloader for hardware platforms. The U-Boot is widely used in embedded designs. The U-Boot supports common processor architectures such as ARM ®, Power Architecture®, Microprocessor without Interlocked Pipeline Stages (MIPS), and x86®. Advent modena m202 blue drivers download for windows 10 8.1 7 vista xp iso. In addition to the bootstrapping. The Das Universal Bootloader (U-Boot) is a firmware/bootloader for hardware platforms. The U-Boot is widely used in embedded designs. The U-Boot supports common processor architectures such as ARM ®, Power Architecture®, Microprocessor without Interlocked Pipeline Stages (MIPS), and x86®. In addition to the bootstrapping. U-Boot Source Code. The current source code is available through the git repository at gitlab.denx.de. Released Versions (and some special snapshots) are available from the DENX file server through HTTPS or FTP. Gitlab.denx.de also hosts the Custodian git trees; you can see the list of all custodian repositories here. I tried to add CONFIGBOOTPARTITIONACCESS and the other options, but They aren't available on u-boot 2009.01. Probably the only one soution is to update my u-boot to 2009.03 version. This is the minimum version with MMC direct access. ROM Monitor version: Texas Instruments X-Loader 1.4.2, U-Boot 2009.01 Micron Package-On-Package MT29C2G48MAKLCJI-6 IT: Comprises of 2Gb NAND x 16 (256MB) and 2Gb MDDR SDRAM x32 (256MB @ 166MHz) Host development system# QNX Momentics 6.4.1 Terminal emulation program (Qtalk, Momentics IDE Terminal, tip, HyperTerminal, etc.) RS-232 serial port.
Acer view 7134e driver download for windows 10 32-bit. Download Acer monitor drivers or install DriverPack Solution software for driver scan and update. Windows XP, 7, 8, 8.1, 10 (x64, x86) Category: monitors. Are you tired of looking for the drivers for your devices? DriverPack Online will find and install the drivers you need automatically. Download DriverPack. Windows 10 Pro Military Standards 2020 Commercial Solutions Brochure New. Get help for your Acer! Identify your Acer product and we will provide you with downloads, support articles and other online support resources that will help you get the most out of your Acer product. Drivers and Manuals. Download File Size: 6.37MB Operating System: Windows 95/Vista/XP/2000 Latest Version / Release Date: N/A / 1996. Windows device driver information for AcerView 7134e. The AcerView 7134e is a monitor that is manufactured by the Acer Company. The images experienced on this monitor are crisp and this is enhanced by its features which are detailed.